Guide
- Installation
- Introduction
- Getting Started
- Declarative Rendering
- Basic Components
- Conditionals and Loops
- Composing with components
- Handling user input
- Animations
- Slots
- Component As Prop
- Device APIs
- React Native
- Ready for more?
- Vue Native Router
- Testing
- Community Libraries
- How does it work?
- How to contribute
- FAQ
Declarative Rendering
At the core of Vue Native is a system that enables us to declaratively render data using straightforward template syntax:
<template> |
<script> |
We have already created our very first Vue Native
app! This looks pretty similar to rendering a template string, but under the hood a lot of work is being done. The data and the native UI elements are now linked, and everything is now reactive.
In addition to text interpolation, we can also bind element attributes like this:
<template> |
<script> |
Here we are encountering something new. The v-bind
attribute. This attribute is called a directive. Directives are prefixed with v-
to indicate that they are special attributes provided by Vue Native, which internal bind with the React Native props. Then-as you may have guessed-they apply special reactive behavior in re-rendering. Here, it is basically saying “keep this element’s title
attribute up-to-date with the message
property on the Vue instance.”
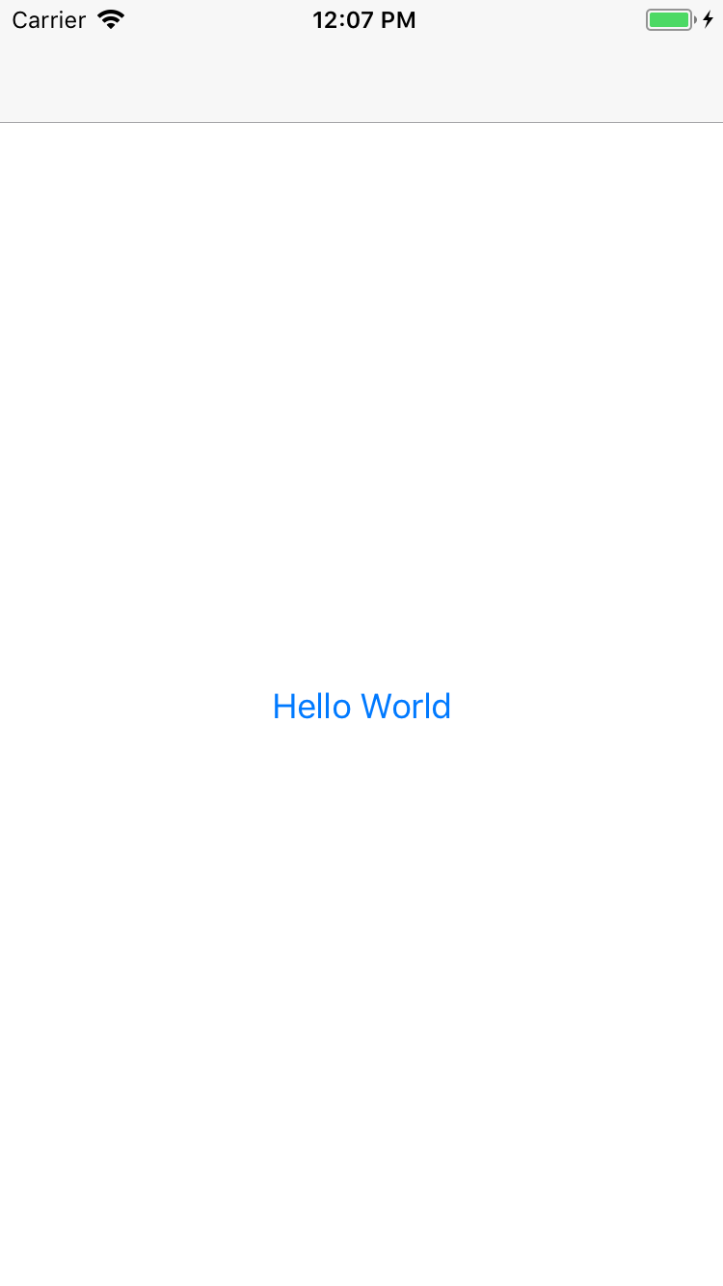
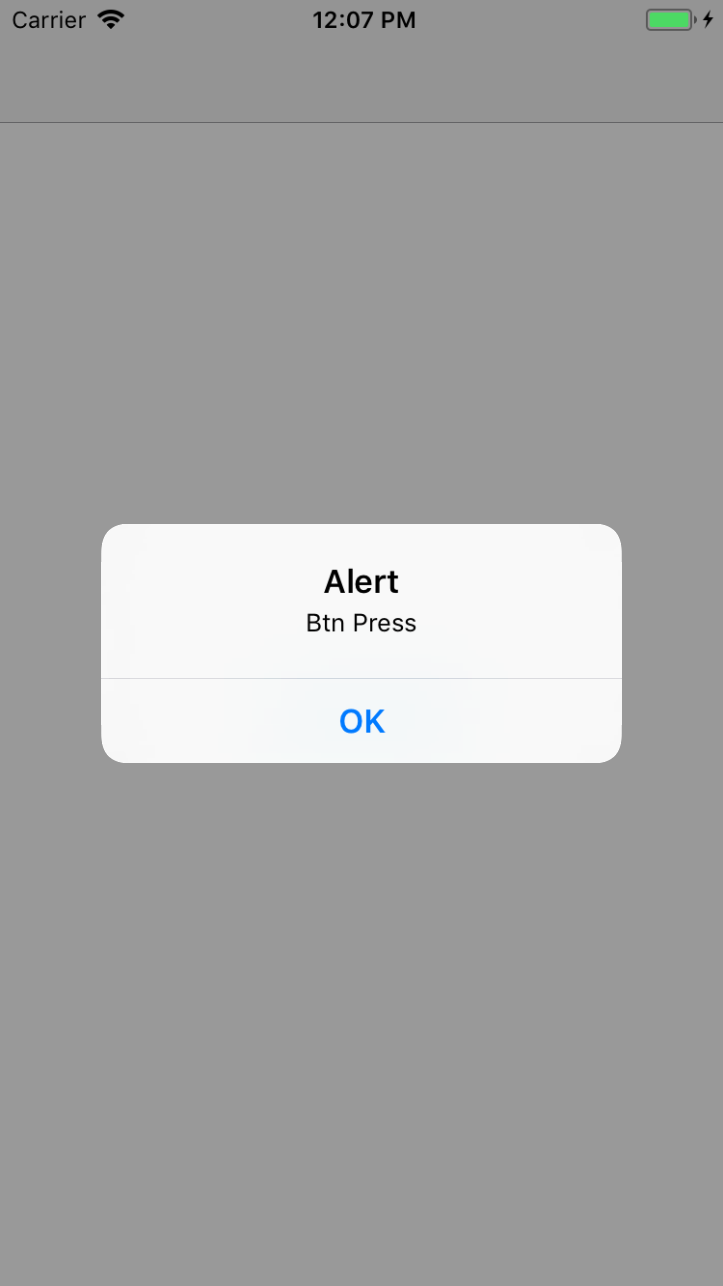