Guide
- Installation
- Introduction
- Getting Started
- Declarative Rendering
- Basic Components
- Conditionals and Loops
- Composing with components
- Handling user input
- Animations
- Slots
- Component As Prop
- Device APIs
- React Native
- Ready for more?
- Vue Native Router
- Testing
- Community Libraries
- How does it work?
- How to contribute
- FAQ
Conditionals and Loops
v-if
Conditionally renders the element or View.
It’s easy to toggle the presence of an element, too! Go ahead and click the button and watch what happens. In Vue, not only can we call methods from event handlers, but we can also run single evaluation statements inline, directly inside the handler.
This is very handy when it comes to something like the v-if
example, you can simply toggle the boolean in our data property inline, instead of having to write a seperate method, then call that method when the event gets fired.
<view> |
<script> |
v-else
Conditionally renders the else block of a
v-if
directive.
The v-else
directive is a secondary(optional) directive that gets used with v-if
. Much like the way an if/else conditional statement works in Javascript, this works similarly in rendering an element, else
redering a different element, based on the value of a data property on our Vue Instance.
Notice how v-else
doesn’t have a parameter input on it? Thats because v-else
is implicit to the most previous v-if
directive in use. Else is will default to an error of missing ‘v-if directive’
<view> |
<script> |
v-show
Conditionally display an element or View.
Much like the v-if directive, v-show acts in a similar way being that they both change the display of the target element or View, but with one key difference.
That difference is how the directive achieves this. v-if
will remove the element from the view completely, where as v-show
keeps the element on the View, and only adjusts the opacity to 0%, or transparent.<view>
<text v-show="seen">Now you see me</text>
<button :on-press="toggleSeen">Click to Toggle</button>
</view>
<script> |
v-for
Renders a list of items using the data from an Array.
This example demonstrates that we can bind data, and dynamically render the UI elements to the View based on the values inside the Array we are looping through. V-for is basically a ForEach loop.
<view class="container"> |
<script> |
<style> |
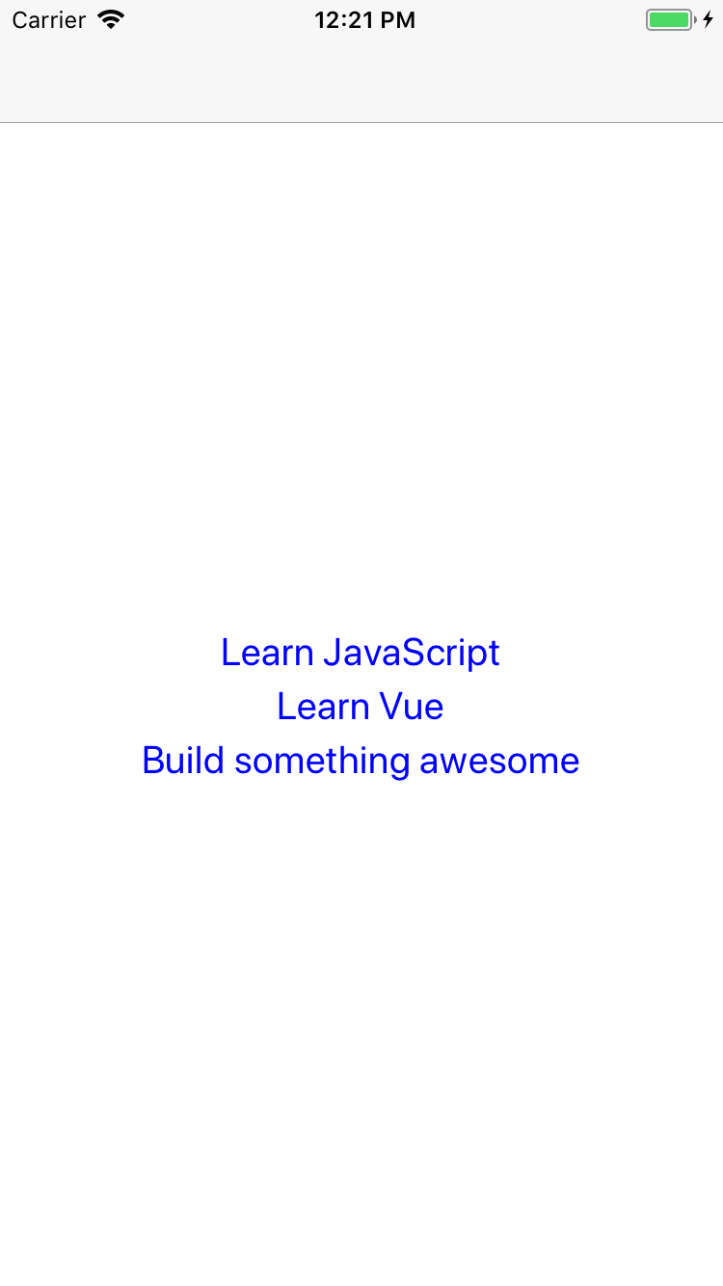